In a digital world where agility and efficiency are paramount, the command line stands as a vital tool, particularly for Linux users. According to a JetBrains survey, an increasing number of developers are turning to Linux-based systems, highlighting the growing relevance of command line proficiency. Our guide, “Conquering the Command Line: Mastering Bash Scripting for Linux Beginners,” aims to demystify the world of Bash scripting, a cornerstone of Linux command line usage. Bash scripting, known for its versatility and power, enables users to automate tasks, manipulate files, and manage systems with ease. This guide is not just about learning commands; it’s about embracing a skill set that enhances problem-solving and automation in the Linux environment. From basic syntax to advanced script writing, you’ll gain a comprehensive understanding that goes beyond mere command execution. Whether you’re a budding developer, a system administrator, or a tech enthusiast, mastering Bash scripting opens doors to a world of efficiency and technical prowess in the Linux ecosystem. This journey through the command line will equip you with the skills to navigate, control, and exploit the full potential of Linux, setting you on a path to becoming a confident and capable Linux user.
Understanding Bash: The Gateway to Linux Mastery
Bash, an acronym for Bourne Again SHell, is more than just a shell; it’s the backbone of command line interaction in Linux systems. This powerful scripting environment is your first step towards mastering Linux, offering unparalleled control and a direct way to interact with the operating system. Whether you’re managing files, running software, or automating tasks, Bash is the tool that ties all your Linux skills together.
Bash Scripting 101: The Core of Linux Command Line
At the heart of Linux command line is Bash scripting, a fundamental skill for any aspiring Linux enthusiast or professional. Bash scripting allows you to automate mundane tasks, streamline complex workflows, and execute powerful commands with precision. For example, a simple script to list all files in a directory might look like this:
#!/bin/bash
# This script lists all files in a directory
echo "Listing all files in the current directory:"
ls
Learning to script with Bash is like learning to speak the language of your computer; it opens up a world of possibilities. From customizing your environment to deploying software, the potential is immense. Various resources, such as LinuxCommand, provide excellent starting points to dive into Bash scripting.
Why Every Linux Beginner Should Learn Bash Scripting
Bash scripting isn’t just a technical skill; it’s a necessity in the modern tech landscape. Understanding Bash means you can navigate, manipulate, and control Linux systems with ease. It’s a critical component for anyone working in IT, software development, system administration, and more. The efficiency and automation capabilities Bash provides are invaluable. As you begin your journey with Bash, remember that each script, each command, is a step towards mastery. Sites like GNU.org offer comprehensive guides to deepen your understanding.
In learning Bash, you’re not just learning commands; you’re learning how to think, plan, and solve problems like a Linux pro. This section is your first step on a path to a deeper understanding and proficiency in the Linux world.
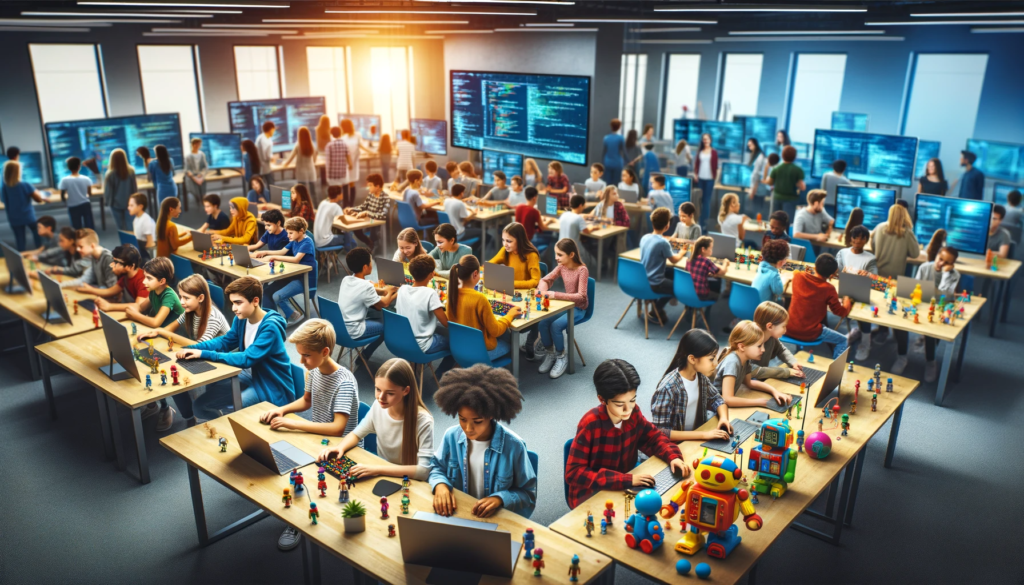
The Linux Command Line Bootcamp: Your First Steps
Embarking on your journey with the Linux command line might seem daunting, but it’s the first step towards tech self-reliance. This bootcamp is designed to take you from novice to knowledgeable by demystifying the essentials of navigation and scripting. Here, you’ll learn the ropes, understand the language, and start speaking directly to your Linux system.
Navigating Through Linux: Basic Commands and Operations
Navigating through Linux begins with understanding its basic commands and operations. Familiarize yourself with commands like ls
for listing directory contents, cd
to change directories, and pwd
to display the current directory. Each command is a building block towards fluency in Linux. For instance:
# Change directory to Documents
cd Documents
# List files in the current directory
ls
# Display the current directory path
pwd
These commands are your toolkit for moving around and understanding the structure of your Linux environment. Resources like Linux Journey can provide further insights and practical examples to solidify your understanding.
Writing Your First Bash Script: A Beginner’s Walkthrough
Writing your first Bash script is a rite of passage into the world of Linux. It’s where you transition from executing single commands to creating your own scripts that can perform complex tasks. Consider this simple script that checks for the presence of a file:
#!/bin/bash
# Check if a file exists
if [ -f "example.txt" ]; then
echo "The file exists."
else
echo "The file does not exist."
fi
This script is a basic example, but it embodies the essence of Bash scripting: automating and making decisions. As you progress, your scripts will grow more sophisticated, handling more complex tasks with ease. Dive deeper into scripting with resources like Bash Academy for comprehensive learning.
Bash and Linux Shell: A Comparative Insight
Delving into the world of Linux shell programming offers a panorama of options, each with its unique features and capabilities. Bash stands out among these as one of the most popular and widely used shells. This section provides a comparative insight, helping beginners understand Bash’s place in the broader context of Linux shells and its practical applications.
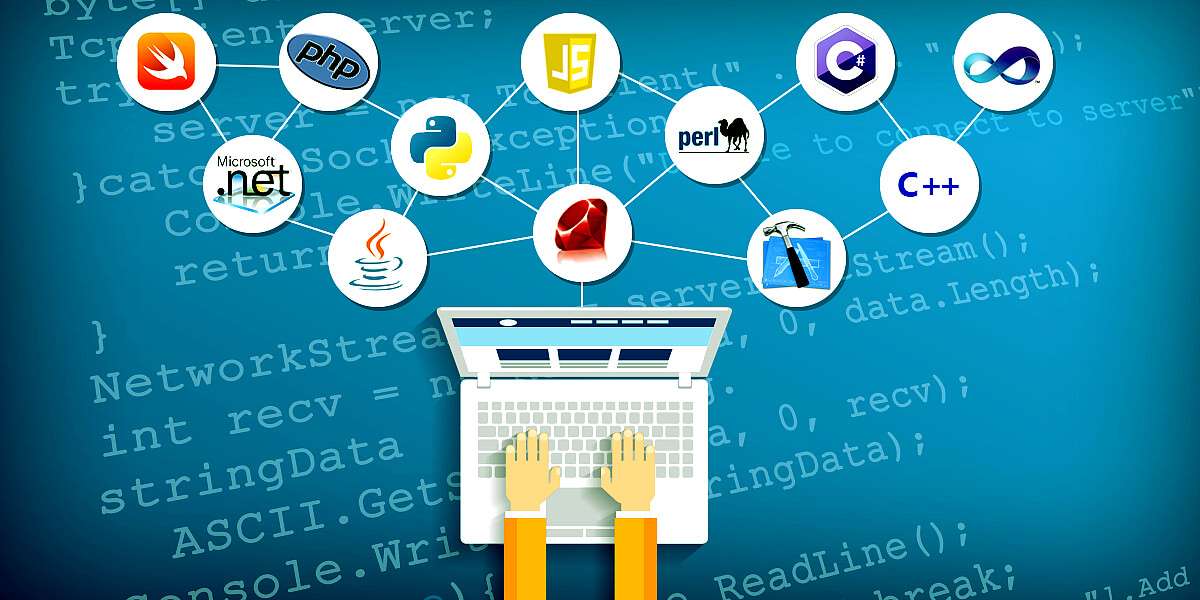
Distinguishing Bash from Other Shells: A Beginner’s Guide
Bash, or Bourne Again SHell, is often the default shell in many Linux distributions. It’s known for its user-friendly scripting language and extensive features. However, other shells like Zsh, Ksh, and Tcsh offer different experiences and functionalities. Zsh, for instance, is renowned for its interactive features and customization options, making it a favorite among power users. On the other hand, Ksh, or the Korn shell, provides advanced scripting capabilities and is often used in commercial environments. Understanding these differences is crucial for beginners to choose the right shell for their needs. Delve into these distinctions with detailed comparisons on TechRepublic.
Practical Applications of Bash in Linux Shell Programming
Bash is not just a command interpreter; it’s a powerful tool for automating tasks, scripting, and even programming. Its syntax is intuitive, and it integrates seamlessly with other Unix utilities. Practical applications range from simple scripts for directory cleanup to complex automation scripts for system administration and development tasks. For instance, here’s a basic Bash script for monitoring disk usage:
#!/bin/bash
# Monitor disk usage
current_usage=$(df | grep '/dev/sda1' | awk '{print $5}')
echo "Current disk usage: $current_usage"
This script demonstrates how Bash can interact with system commands and process information, a fundamental aspect of Linux shell programming. As you explore further, you’ll find Bash’s utility in various domains, from system administration to development environments. Enhance your skills with advanced tutorials and real-life examples from Linux.com.
By understanding both the theoretical and practical aspects of Bash compared to other shells, beginners can make informed decisions and better harness the power of Linux shell programming. This insight is just the beginning of a journey into a more efficient and automated computing experience.
Overcoming Obstacles: Common Challenges for Bash Beginners
Every journey has its hurdles, and the path to Bash proficiency is no exception. Beginners often encounter a series of common challenges that can seem daunting at first. Understanding these obstacles and how to overcome them is crucial for a smooth learning experience. This section delves into typical issues like syntax errors and permission problems, offering solutions and insights to help you navigate these early challenges with confidence.
Syntax Errors and Solutions: Debugging Your First Scripts
One of the first roadblocks many new Bash users face is syntax errors. These errors can range from missing semicolons to incorrect command usage. For instance, a common mistake is forgetting to close a statement or mistyping a command. Here’s an example of a simple syntax error:
#!/bin/bash
echo "Hello World # Missing closing quote
To overcome these, start by reading error messages carefully; they often provide clues about what’s gone wrong. Additionally, use tools like ShellCheck, a powerful online tool that analyzes your scripts and suggests fixes for common mistakes. Further, familiarize yourself with Bash’s syntax and best practices by visiting Linux Academy, which provides extensive resources for beginners.
Permission and Execution: Understanding Linux File Permissions
Another common hurdle is understanding and managing file permissions, which determine who can read, write, or execute a script. Beginners might encounter “Permission Denied” errors when trying to execute a script. This is often due to inadequate execution permissions. To change a script’s permissions to make it executable, you can use the chmod
command like so:
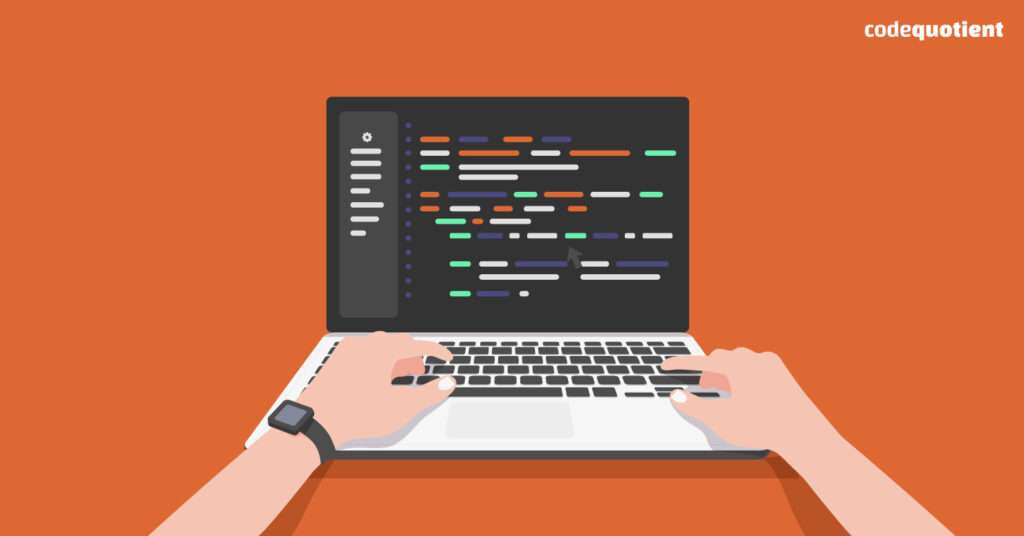
chmod +x myscript.sh
Understanding the Linux permission system is vital for running and managing scripts securely. Websites like Linuxize offer detailed guides on Linux file permissions and how to use them effectively. Additionally, explore forums and communities like Stack Overflow for real-world problems and solutions related to Bash scripting and file permissions.
By addressing these common challenges, beginners can move past the initial frustrations and towards a more profound understanding and appreciation of Bash scripting. This knowledge is not just about solving problems but also about building a solid foundation for future learning and development in the Linux environment.
Intermediate Bash Scripting: Enhancing Your Skills
Progressing from basic to intermediate Bash scripting marks a significant milestone in your journey. It’s about enhancing your skills, diving deeper into the mechanics, and understanding the subtleties that make Bash a powerful tool for automation and problem-solving. This stage is where you begin to move away from simple commands and towards complex, efficient scripts that can handle a variety of tasks with ease.
Control Structures and Loops: Automating with Bash
Control structures and loops are the backbone of automation in Bash scripting. They allow you to make decisions and repeat tasks efficiently. For instance, if-else
statements enable you to execute commands based on conditions, while loops like for
and while
let you repeat tasks until certain conditions are met. Here’s a basic example of a for
loop in Bash:
#!/bin/bash
# A simple for loop
for number in 1 2 3 4 5
do
echo "Number is: $number"
done
This script will print numbers from 1 to 5, demonstrating how loops automate repetitive tasks. For a more comprehensive understanding, explore resources like Bash Guide for Beginners which offers in-depth explanations and examples.
String Manipulation and Scripting Functions: The Building Blocks
String manipulation and functions are essential tools in any scripter’s arsenal. They allow you to handle text, one of the most common elements in scripting, and create reusable code blocks. Bash provides a variety of ways to manipulate strings, from simple concatenation to complex pattern matching. For example:
#!/bin/bash
# Basic string manipulation
greeting="Hello"
user="User"
echo "$greeting, $user!"
This script demonstrates basic string concatenation. For more complex operations, sites like Bash Hackers Wiki provide extensive guides and examples. Functions in Bash, much like in other programming languages, allow you to encapsulate code for a specific task, making your scripts more modular, readable, and maintainable.
By mastering these intermediate concepts, you enhance your ability to create powerful, efficient scripts that can automate tasks, process data, and make your life easier. Remember, practice is key; the more you script, the more proficient you’ll become. Continue to expand your knowledge, experiment with new ideas, and utilize the vast array of online resources available to intermediate Bash scripters.
Advanced Bash Scripting: Unlocking Full Potential
Reaching the advanced stages of Bash scripting is about more than just writing scripts; it’s about unlocking the full potential of what you can achieve with this powerful tool. Advanced Bash scripting involves a deep understanding of the shell’s capabilities and the integration of various utilities to manipulate data, automate complex tasks, and optimize performance. It’s here that you transition from following instructions to creating sophisticated solutions tailored to specific problems.
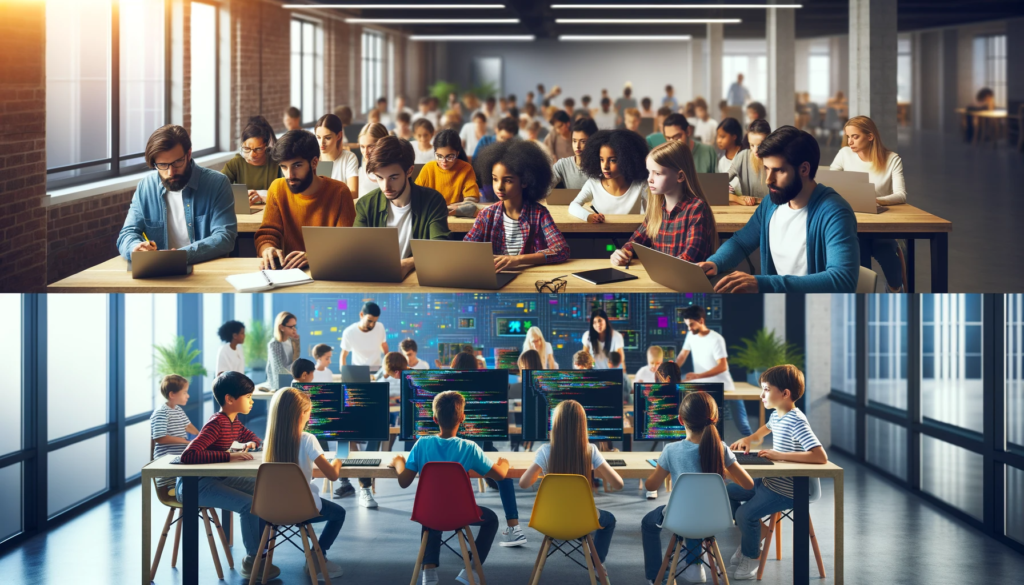
Sed, Awk, and Grep: Text Processing Power Tools
The commands above are amongst the most powerful text processing tools in Unix-like systems. Sed, the stream editor, is perfect for modifying text in a script. Awk is a complete text processing language ideal for transforming data and generating reports. Grep, known for finding text patterns, is indispensable for searching through large volumes of data. A simple example of using grep is as follows:
grep 'error' /var/log/system.log
This command searches for the word ‘error’ in the system log. Complex tasks might combine these tools, leveraging their unique strengths to process data efficiently. Websites like GNU Manuals provide comprehensive guides to mastering these utilities.
Scripting Like a Pro: Advanced Techniques and Best Practices
Advanced Bash scripting is also about adopting best practices and techniques that ensure your scripts are robust, efficient, and maintainable. This includes using functions to modularize code, employing error handling to make scripts reliable, and optimizing performance through efficient use of resources. Here’s a snippet demonstrating a function and error handling:
#!/bin/bash
# A sample function with error handling
function check_file {
if [[ -f "$1" ]]; then
echo "File exists."
else
echo "Error: File does not exist." >&2
exit 1
fi
}
check_file "/path/to/file"
This script checks if a file exists and handles the situation where it doesn’t. Advanced scripters know the importance of such practices and continually refine their scripts for maximum effectiveness. For more on best practices, visit Bash Guide, which offers a wealth of information.
As you explore the advanced aspects of Bash scripting, remember that it’s a journey of continuous learning and improvement. The more you experiment and tackle real-world problems, the more proficient you’ll become, unlocking the full potential of what you can achieve with Bash.
Practicing Bash: Pathway to Mastery
Practicing Bash is not just about learning; it’s about doing, experimenting, and constantly challenging yourself with new tasks. This practical approach is the pathway to mastery, transitioning you from understanding concepts to applying them in real-world scenarios. By engaging with exercises, projects, and the community, you solidify your knowledge and develop a profound, intuitive understanding of how Bash scripting can solve complex problems and streamline tasks.
From Exercises to Real-World Projects: Applying Your Knowledge
Starting with exercises, move gradually to more complex, real-world projects to apply your Bash scripting knowledge. Begin with simple tasks like file management and system updates, then progress to automating backups or data analysis tasks. Engaging with real-world projects not only reinforces what you’ve learned but also provides a sense of accomplishment and practical utility. For instance, you might create a script to organize your downloads folder automatically, demonstrating how Bash can bring efficiency to everyday tasks. Websites like OverTheWire offer practical exercises that mimic real-world challenges, helping to bridge the gap between theory and practice.
Continuous Learning: Resources and Communities for Growth
Mastery in Bash scripting, as with any skill, comes from continuous learning and engagement with the community. Online forums, user groups, and websites are treasure troves of information, offering tutorials, discussions, and insights that can propel your understanding forward. Engaging with communities on platforms like Stack Overflow or Reddit’s r/bash can provide support, inspiration, and a sense of belonging. Additionally, keeping abreast of the latest updates and features in Bash through resources like Bash Hackers Wiki ensures that your skills remain sharp and relevant.
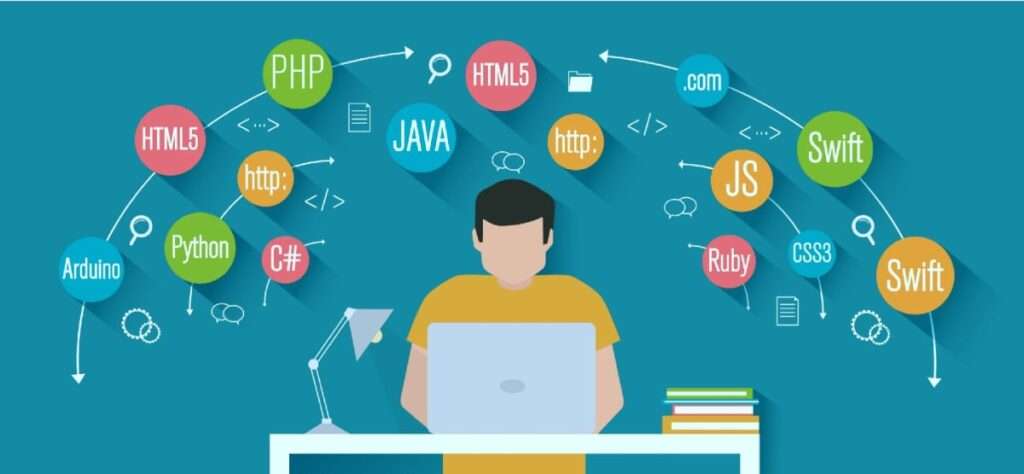
Conclusion
As we wrap up this comprehensive journey through “Conquering the Command Line: Mastering Bash Scripting for Linux Beginners,” it’s clear that the command line is more than just a tool; it’s a gateway to unlocking the full potential of Linux. From the basics of Bash scripting to the advanced techniques that seasoned pros use, you’ve been equipped with the knowledge and skills necessary to navigate this powerful environment. Remember, mastering Bash scripting is not just about understanding commands; it’s about developing a mindset that sees solutions in challenges and opportunities in every script.
Your curiosity and determination have brought you this far, and as you stand on the threshold of command line mastery, it’s your ongoing practice and engagement with the community that will continue to propel you forward. Whether you’re automating tasks, managing systems, or simply exploring the vast capabilities of Linux, Bash scripting is your steadfast companion.
Now, as you move from learning to doing, let the principles and techniques you’ve learned here ignite a spark of creativity and efficiency in your work. Continue to experiment, learn, and grow. The world of Linux and Bash scripting is vast and ever-evolving, and your journey is just beginning.
For those in search of the latest insights, trends, and buzz, you’re in the right place at ItzNews. Explore our curated list of must-read articles:
- 🌐 For a look into the future of technology: Werner Vogels’ Tech Predictions for 2023: A Deep Dive
- 💧 Interested in enhancing your well-being? Don’t miss The Power of Water: Harnessing its Benefits for Optimal Human Health
- ⚽ Sports aficionado? Stay updated with Premier League transfer news featuring Cristiano Ronaldo and top clubs
Dive into these topics and more to stay informed, enlightened, and entertained.